Minimal WebApi with ASP.NET Core (Visual Studio 2022)
- 10.11.2022
- 42
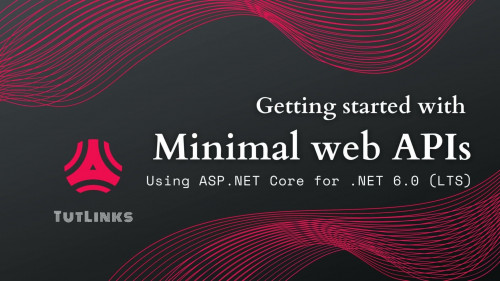
Minimal WebApi with ASP.NET Core (Visual Studio 2022)
Published 09/2022
MP4 | Video: h264, 1280x720 | Audio: AAC, 44.1 KHz, 2 Ch
Genre: eLearning | Language: English + srt | Duration: 36 lectures (2h 25m) | Size: 804 MB
Beginner to advanced course for professionals and college students
What you'll learn
Minimal WebApi of C# ASPNET Core (Visual Studio 2022 and later)
GET, POST, PUT and DELETE various types covered
Calling from desktop C++ App, C# Console, C# WPF Apps
Use of HttpRepl
Parameter Binding to QueryString, Header and Custom Bindings
FileUpload with WebApi
JWT Server and Client Side Authentication
Basic Authentication
Social Media based Authentication (Google)
Requirements
Some knowledge of C#
Some knowledge of Entity Framework C#
Description
This course is a brief course that teaches minimal web-api of ASP.NET Core. Explanation is in a simple language. Downloads have been provided with almost every chapter. Tutorials have been kept brief and to-the-point. Audio is crystal clear.
Getting Started with Web Api
This tutorial starts with a beginner's introduction to the concept of web api. After that we create a basic structure of a project that will help us learn about various types of web api - which will be serially covered in the next coming tutorials.
Minimal GET WebApi with No Parameters
In this tutorial we shall add a web api that allows us to get all the records of a table. Please do note, however, that such a query is never done in a real project - it is invariably qualified with some paramaters that return a lesser number of records.
Minimal GET WebApi with a Parameter of Numeric Type
In the previous tutorial we learned how to write a web api that accepts no parameters. We executed it to return all the records of a table. In this tutorial we shall add another web api that accepts a parameter and returns data on the basis of that parameter.
Minimal GET WebApi to Find a Record by Id
In this tutorial we shall add a web api of GET type that takes an ID as a parameter and returns a record for that parameter.
Using Postman to Run WebApi
Postman is a software that can be used to easily and conveniently run and execute web api. It can be installed as a desktop application. The software offers a lot of functionality, but we shall be using a small part of that - relevant to just our web api needs.
Minimal POST WebApi for Creating a new Record
A web api of the POST type is used to create a new record. For example, if we have to create a new record of a doctor, then we have to use a POST api to send the relevant fields for creating a record.
Minimal PUT WebApi for Updating a Record
An Http PUT request is used to update an existing record. For this, a client has to send the unique id of the record along with the entire updated record. Partial updates are not done with a PUT type of Web Api. Partial updates are, rather, supported by an HTTP PATCH request. But that is not the topic of this tutorial. Today we shall focus on the Http Put type of Web Api.
Minimal DELETE WebApi for Deleting a Record
An HTTP DELETE request is used for deleting a record. The Server sends a 200 OK response on successful deletion alongwith the item that was deleted. Alternatively, it can send 204 No Content if the deleted item is, or cannot be included in the response. Let us see the implementation in this tutorial!
Over-Posting in WebApi and a Solution with Data Transfer Object
We have been working with a model class consisting of three properties - id, Name and Fees. All the three properties have participated and have been exposed in json communication through every Web Api that we have discussed and written in the past tutorials of this chapter. This is called over-posting. But what if there were a property such as an email address that we didn't want exposed? This tutorial presents a solution through the concept of a Data Transfer Object.
Preventing Over-Posting of a Collection in a WebApi
If an API has to return a collection of records, and if we do not want entire objects transmitted, then we can use a Data Transfer Object to return a small subset of properties, just-the-required subset. For a primer on over-posting, please refer the previous tutorial "Over-Posting in WebApi and a Solution with Data Transfer Object".
DTO Object as Argument and Return in a WebApi
This tutorial explains how to modify a POST type of WebApi so that it doesn't over-post data. A POST WebApi receives a data object as a parameter, and then adds it to a database, and finally it returns an HTTP 201 Created response alongwith the json for the newly added object. We have already covered this in one of the previous tutorials and for a primer on over-posting, please refer a previous tutorial "Over-Posting in WebApi and a Solution with Data Transfer Object".
Calling a GET WebApi from a C++ WinRT App
This tutorial explains how a GET web api can be called from a C++ WinRT application. I have chosen WinRT type because it contains a lot many pre-written functions that simplify internet access and json parsing. I won't be able to explain the details of a WinRT setup, so I have already created a WinRT console application that connects to our web api, and then obtains a string from the application, and then parses the json contained in that string. The c++ project has been provided in the downloads attached to this course.
Calling a POST WebApi from a C++ WinRT App
This tutorial explains how a POST web api can be called from a C++ WinRT application. I have created a WinRT console application that connects to, and posts a json string to a POST web api, and then obtains a string response from the application, and then parses the json contained in that string. The c++ project is available in your downloads.
OpenAPI and Swagger for Web API Documentation
Swagger makes it easy to document the various Web Api exposed by your project. The summary of your API is maintained in a json based file called openapi.json. This document is the main document. It is an intermediate document that is used to create a web page called SwaggerUI - which shows all your web api in a colorful, human readable interface. Each web api can be immediately run and tested. It turns out that SwaggerUI is much more easier as compared to the Postman software.
Configuring and Using Swagger to Run WebApi
Let us now configure our WebApi project to add support for SwaggerUI. As we told earlier, SwaggerUI is provided by both SwashBuckle and NSwag. We can use either of the two - and I shall be using SwashBuckle.
Call a GET Web API From a C# WPF Application
In this tutorial we shall learn how to call the various types of WebApi from a C# WPF application. I have already created a WPF application that obtains a list of records from our web api project and renders them in a grid. This is a GET web api request that queries a collection of records. The same WPF window has a form for creating a new record - and this is done with a call to the POST web api. When a user clicks a record on the WPF window, a dialog pops up that allows the user to update the record. This will be a call to the PUT Web Api. The same dialog has a delete button that makes a call to the DELETE Web Api.
Call a POST Web API From a C# WPF Application
This tutorial continues from the previous tutorial. We have discussed most of the parts there. So please go through the previous tutorial for a continuity. Now we shall call a POST WebApi from our Wpf Application, and create a new record.
Call a PUT and DELETE Web API From a C# WPF Application
As you must have already seen in the previous tutorials, we have a WPF application that obtains a list of records from our ASPNET Core web api application, and shows them in a WPF datagrid. When a row of the datagrid is clicked, a dialog pops-up that shows a form for editing the record. The save button for this form is wired to a click event handler that makes a PUT call to a web api and updates the record. A delete button on the same form causes a call to a DELETE web api.
Introduction to HttpRepl for Testing Web Api
Http Repl is a command-line tool for testing web api. This tool targets the ASP.NET Core environment. Let's have a look at a brief introduction to this tool. We will also learn how to activate it in visual studio. And in the coming tutorials we shall use it to make a few web api calls.
Connecting HttpRepl to a Web Api Endpoint
Before we can execute and test any web api, we have to establish a connection betweenhttprepl and our web server. If you are following this chapter, then you must have observed that each of our route templates starts with the segment "doctor". Technically, it is called an end-point. The objective of this tutorial is to learn how to connect to a server, and how to get a list of available endpoints, and how to finally navigate to a specific endpoint - which is "doctor" in our case. In the coming tutorials we shall make calls to the various types of web api that we have already added to our web api project.
Testing a GET Web Api with HttpRepl
Now its time to usehttprepl for testing our web api. To begin with, we shall test a GET api. I shall assume that yourhttprepl is installed and ready. If things appear difficult, then you may have to go through the previous two tutorials for learning the basics ofhttprepl.
Testing a POST Web Api with HttpRepl
In this tutorial we shall test a POST Web Api - and create a new record. So we shall have to first establish a connection betweenhttprepl and our web server, and, then we will have to post the json for the new record that we have to create on the server. Let's see how!
Using a static class for Route Handlers in a Minimal WebApi
So far, we have been writing all our minimal web api in the program.cs file. But this can become un-manageable and un-readable in a full blown project. We need a scheme for organizing our web api so that they can stay in a class of their own, inside a file of their own. We can even have a possibility of multiple classes - with each class containing all the related web api - those of a doctor in one file, whereas those of a patient in another. In this tutorial we have a brief look at the various possibilities, and finally I show an implementation of the scheme that I personally prefer.
[FromQuery] Parameter Binding of a QueryString Variable
We have been obtaining our web api parameters from route templates. A route is not the only source of parameters - in fact the parameters can be obtained from a query string, header values and from the body of the request also. In this tutorial we examine how to obtain the parameters from a query string variable, and how to set the parameter as optional.
[FromHeader] Parameter Binding of a Request Header
Parameters of a web api can be obtained from the values in a request header also. For this an attribute called "FromHeader" is used to decorate a parameter, and it provides an easy access to the value contained in the header of that name. In this tutorial we shall learn how to bind a header to a web api parameter. For continuity please go through the previous tutorial where we saw how to bind a query string variable to a parameter.
Special Types of Implicit Parameter Bindings
ASP.NET Core provides certain "special" parameters that are always available to an api handler. These parameters are "utility" parameters like HttpContext, HttpRequest, HttpResponse, CancellationToken and ClaimsPrincipal. They do not need any attribute decorations like those for the query-string, header, body and route segments.
Customized Binding of API Parameters
Consider a web api that extracts parameters x, y and z from its query string and then uses these values to construct a Point object, say for example, by passing these data to a constructor of the Point class. This program can be made more readable by passing a single string of a comma separated list of three numbers, and then have that string directly parsed into a Point object. This technique of customized binding is the subject matter of this tutorial.
BindAsync for Customized Binding of WebApi Parameters
BindAsync is a second method for custom binding of parameters. The first one - TryParse - is good for cases where the items are available in a single string - most ideally as in a query string, or a header value, or a route segment. However, if need a greater control over the binding process, then a more robust parameter such as the HttpContext is more suitable - and the BindAsync method does exactly that. It provides a signature that contains HttpContext as a parameter.
File Upload with a WebApi
In this tutorial we examine how to write a web api that accepts an uploaded file and saves it to a server. We shall also present a C# console application that will post a file to that web api. Let's see how!
Basic Authentication in WebApi
Basic Authentication is not encourged by ASP.NET Core because login id and password are sent as plain text in the request header - it's vulnerable to XSRF also. So there are no readymade classes like we have for cookies based authentication. But what if your project still needs to allow basic authentication? Perhaps because your security requirements are not of extreme cutting-edge type? For that case we present a simple solution that helps you protect a web-api with basic authorization. It can be a lot safer with Basic Authentication if your communication is overhttps, and even better if XSRF measures are taken, which is beyond the scope of this tutorial.
HandleAuthenticateAsync and Basic Authentication in WebApi
The recommended way of implementing a custom authentication scheme is by deriving a class from AuthenticationHandler and implementing the HandleAuthenticateAsync function. This makes the code systematic because the authentication and header parsing code now moves to a dedicated class. This approach also allows us to include multiple authentication schemes in the same project - for example you might want one web api to be authorized through basic authentication, and another one through a JWT based authentication, and yet your razor pages through a cookie based authentication. In this tutorial we implement the basic authentication scheme.
JWT Authentication in WebApi
JWT stands for JSON Web Token. In its simplest form, JWT authentication is a two step process. First a client obtains a JWT token from the server. This token is signed with a secure key and encrypted with a standard encryption algorithm. It has an expiry date, and it contains a list of claims such as the user-id, user-role, his email, etc., The client obtains authorization to a web api by sending this token in an authorization header. In this tutorial we shall see a server side implementation of the code for generating a JWT token, and of protecting a web api.
C# Console App Connectivity for Basic and JWT Authentication
In this tutorial we present a C# console application that connects to the web api that we have done in the previous tutorial. This app will first obtain a JWT Token by sending it's login credentials in a basic authorization header, and then it will use that token in a bearer header to obtain authorization to the second api protected by JWT Authorization. Please go through the previous turorial where we have explained both the web apis.
Creating a Google OAuth App for Authentication
Social media based authentication requires us to create an oauth app on a social media platform such as google, facebook, twitter, github. This app provides us a client id and a client secret key for use in various classes that provide social media integration. In this tutorial we have a walkthrough for creating an oauth app on the google cloud console. Steps for other social media are similar - so you can take them as an exercise.
Social Media Authentication in WebApi
WebApi can be protected through social media authentication also. The first step is to obtain a client id and a client secret by creating an app on a social media platform. Then these values are used to configure authentication services for that platform. In this tutorial we present the sequence of steps required to gain authorization to a web api protected by google authentication.
Who this course is for
Beginners and Students (language used is simple, crude)
Homepage
https://anonymz.com/?
https://www.udemy.com/course/minimal-webapi-with-aspnet-core/
Screenshots
#
https://nitroflare.com/view/D5DE0CAA7D11C6C/Minimal_WebApi_with_ASP.NET_Core_%28Visual_Studio_2022%29.rar
https://rapidgator.net/file/4470ba445610ca2c858548b692a47327/Minimal_WebApi_with_ASP.NET_Core_(Visual_Studio_2022).rar.html
https://rapidgator.net/file/4470ba445610ca2c858548b692a47327/Minimal_WebApi_with_ASP.NET_Core_(Visual_Studio_2022).rar.html